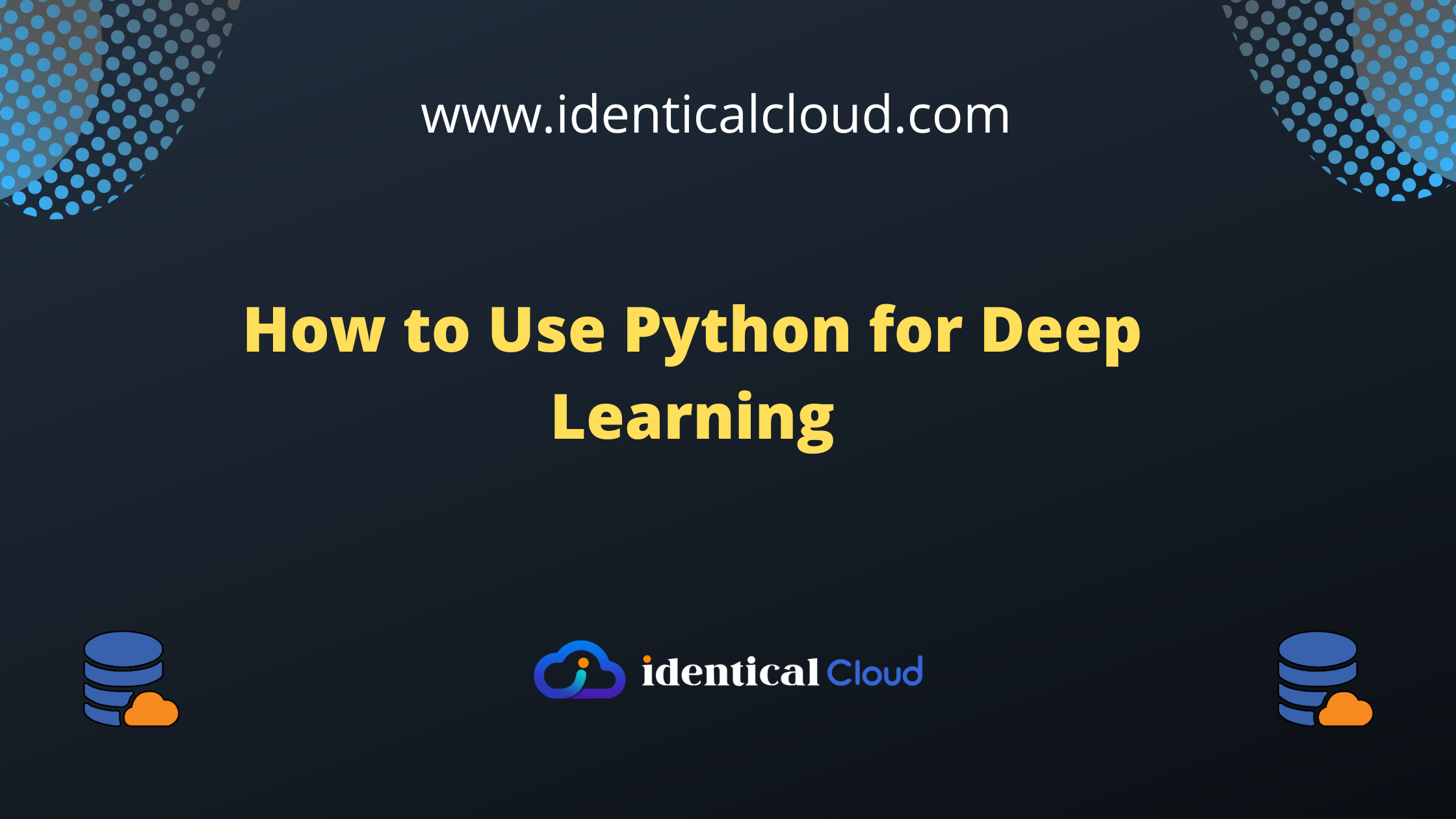
How to Use Python for Deep Learning
How to Use Python for Deep Learning
Python has become the go-to language for deep learning due to its powerful libraries, vast community, and beginner-friendly syntax. This blog post will guide you through the essential steps of using Python for deep learning, equipping you with the knowledge to unleash its potential for your projects.
Why Python for Deep Learning?
Python’s popularity in the deep learning community stems from several key factors:
- Ease of Learning: Python’s simple and readable syntax makes it easy for beginners to grasp fundamental concepts quickly.
- Vast Ecosystem: Python offers a rich ecosystem of libraries and frameworks tailored for deep learning, such as TensorFlow, PyTorch, Keras, and scikit-learn, which provide efficient tools for building and training neural networks.
- Community Support: Python has a large and active community of developers and researchers who contribute to the development of deep learning tools, share tutorials, and offer support through forums and online communities.
- Flexibility: Python’s versatility allows deep learning practitioners to seamlessly integrate their models with other data processing libraries, visualization tools, and web frameworks.
Getting Started with Python for Deep Learning
1. Choose a Deep Learning Framework
There are several deep learning frameworks available in Python, each with its own strengths and capabilities. Some popular frameworks include:
- TensorFlow: Developed by Google Brain, TensorFlow is widely used for building and deploying deep learning models in production environments.
- PyTorch: Developed by Facebook’s AI Research lab, PyTorch is known for its dynamic computation graph and intuitive API, making it popular among researchers and practitioners.
- Keras: Keras is a high-level neural networks API, written in Python and capable of running on top of TensorFlow, Theano, or CNTK. It offers a user-friendly interface for building and training deep learning models.
2. Install Required Libraries
Once you’ve chosen a deep learning framework, you’ll need to install it along with other necessary libraries. You can use Python package managers like pip or conda to install libraries and dependencies:
pip install tensorflow
pip install torch torchvision
pip install keras
3. Learn the Basics of Deep Learning
Before diving into complex deep learning projects, it’s essential to understand the basic concepts and principles behind neural networks, including:
- Feedforward Neural Networks
- Convolutional Neural Networks (CNNs)
- Recurrent Neural Networks (RNNs)
- Loss Functions and Optimization Algorithms
- Data Preprocessing and Augmentation
There are numerous online resources, tutorials, and courses available to help you learn these concepts, including the official documentation and community forums for your chosen deep learning framework.
4. Build and Train Your First Model
After gaining a basic understanding of deep learning concepts, you can start building and training your first neural network model. Begin with simple projects and gradually increase the complexity as you gain experience.
Here’s a simple example of building a feedforward neural network using TensorFlow:
import tensorflow as tf
from tensorflow.keras import layers, models
# Define the model architecture
model = models.Sequential([
layers.Dense(64, activation='relu', input_shape=(784,)),
layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=10, batch_size=32)
5. Experiment and Iterate
Deep learning is an iterative process, and experimentation plays a crucial role in improving model performance. Experiment with different architectures, hyperparameters, and optimization techniques to find the best configuration for your specific task.
Python’s simplicity, versatility, and rich ecosystem of libraries make it an ideal choice for deep learning projects. By leveraging popular deep learning frameworks like TensorFlow, PyTorch, and Keras, developers and researchers can build powerful neural network models to tackle a wide range of tasks, from image recognition to natural language processing.