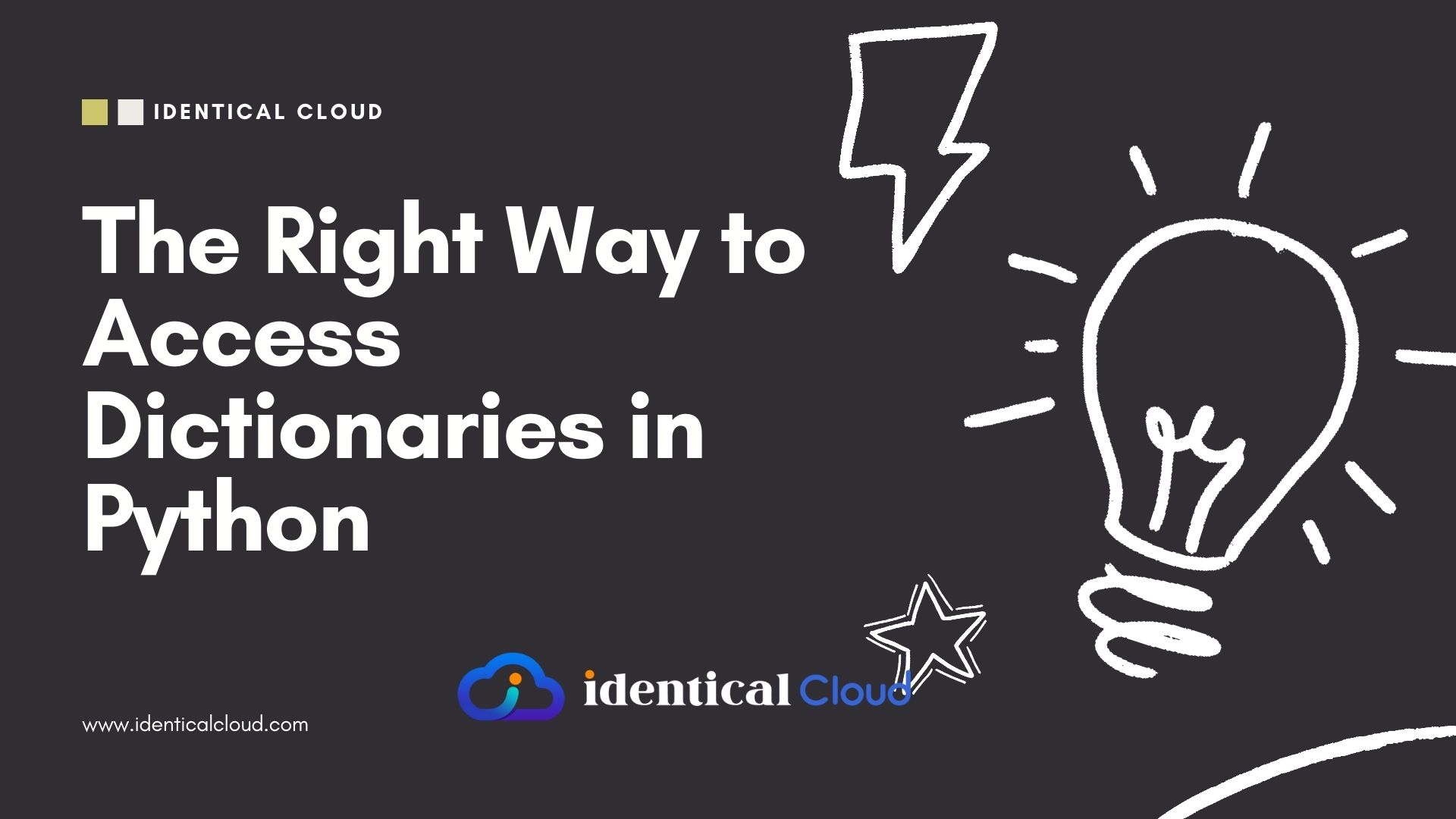
The Right Way to Access Dictionaries in Python
The Right Way to Access Dictionaries in Python
Python dictionaries are a powerful tool for storing and organizing data, offering flexibility and fast lookups. If you’re working with Python, understanding how to access dictionary elements effectively is a crucial skill. Let’s explore the essential techniques to retrieve and manipulate data within your dictionaries.
Fundamentals: Keys and Values
- Dictionaries consist of key-value pairs. The key is a unique identifier within the dictionary, while the value is the associated data.
- Keys must be immutable types (e.g., strings, integers, or tuples). Values can be of any data type, including lists or other dictionaries.
Methods of Access
1. Square Bracket Notation (Retrieving a Value):
- The most common method, using square brackets with the key inside:
my_dict = {"name": "Alice", "age": 30}
name = my_dict["name"] # Accessing the value associated with "name"
- If the key doesn’t exist, it will raise a
KeyError
exception.
2. The .get()
Method (Safe Retrieval):
- Retrieve values safely without risking a
KeyError
:
value = my_dict.get("name") # Returns the value if "name" exists
value = my_dict.get("city", "None") # Returns "None" if "city" doesn't exist
- Allows you to specify a default value in case the key is not found.
3. Checking for Key Existence:
- Use the
in
andnot in
operators:
if "name" in my_dict:
print("Name exists in the dictionary")
4. Iterating Through Dictionaries:
- Loop over keys:
for key in my_dict:
print(key)
- Loop over values:
for value in my_dict.values():
print(value)
- Loop over both keys and values:
for key, value in my_dict.items():
print(f"Key: {key}, Value: {value}")
Remember:
- Choose the right method based on whether you need to handle potential missing keys.
- Always be mindful of
KeyError
exceptions when using square bracket notation. - Iterating with
.items()
is a great way to work with both keys and values simultaneously.
With these techniques in your arsenal, you’ll be a dictionary-wrangling pro in no time!