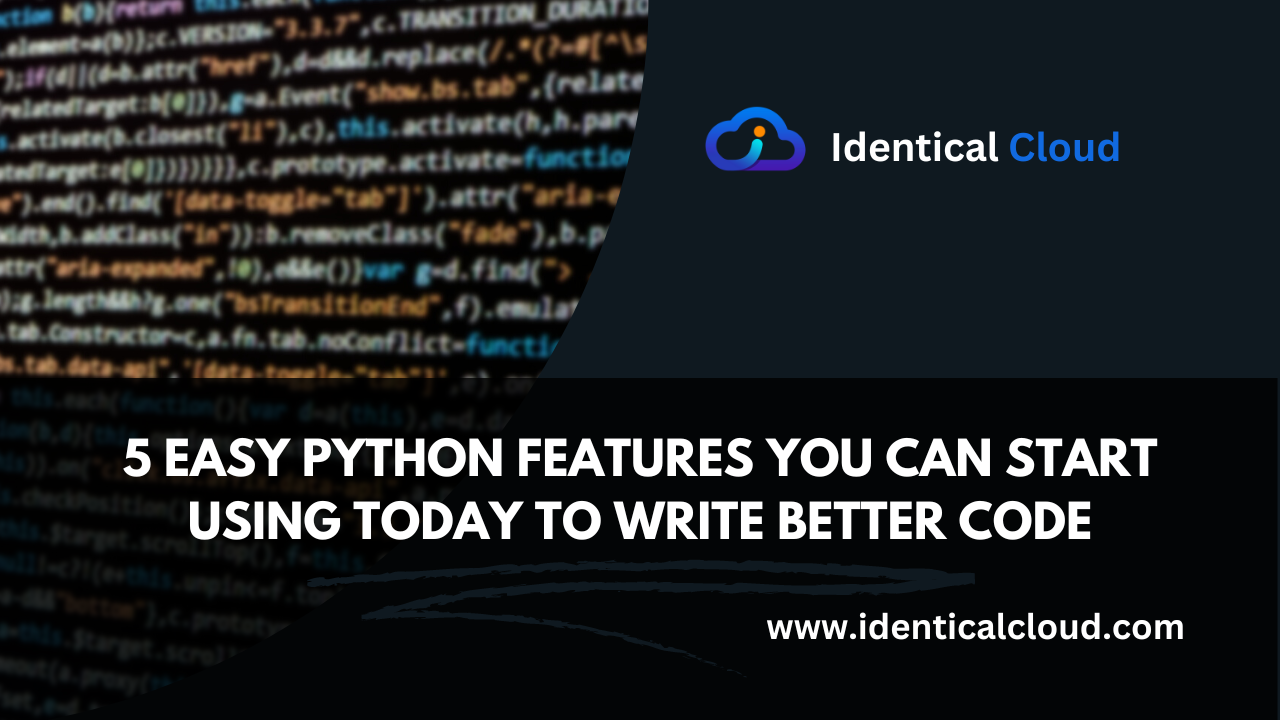
5 Easy python features you can start using today to write better code
5 Easy python features you can start using today to write better code
Python is a versatile and powerful programming language known for its simplicity and readability. While it’s already beginner-friendly, there are several features and techniques that can help you take your Python coding skills to the next level.
In this blog, we’ll explore five easy-to-implement Python features that can instantly enhance your code quality and efficiency.
List Comprehensions
List comprehensions are a concise and elegant way to create lists in Python. They allow you to generate new lists by applying an expression to each item in an existing iterable (e.g., a list, tuple, or string) and filter the results if needed. This feature not only reduces the amount of code you need to write but also improves readability.
Example:
# Traditional approach
squared_numbers = []
for num in range(1, 6):
squared_numbers.append(num ** 2)
# Using list comprehension
squared_numbers = [num ** 2 for num in range(1, 6)]
Generator expressions
Generator expressions are similar to list comprehensions, but they do not actually create a list. Instead, they generate the values one at a time, which can be more memory efficient. For example, the following code creates a generator expression that generates the squares of the numbers from 1 to 10:
squares = (x * x for x in range(1, 11))
You can iterate over a generator expression just like a list:
for square in squares:
print(square)
Decorators
Decorators are a powerful way to add functionality to functions without modifying the original function code. For example, the following decorator adds a logging function to all functions in a module:
def log_function(func):
def wrapper(*args, **kwargs):
print("Calling function:", func.__name__)
return func(*args, **kwargs)
return wrapper
@log_function
def square(x):
return x * x
print(square(10))
This code will print the following output:
Calling function: square 100
Context Managers (with Statements)
Context managers, implemented using the with statement, help manage resources like files, sockets, and database connections efficiently. They ensure proper allocation and deallocation of resources by encapsulating the setup and teardown operations, leading to cleaner and more reliable code.
Example:
# Traditional approach
file = open('example.txt', 'r')
try:
content = file.read()
finally:
file.close()
# Using context manager
with open('example.txt', 'r') as file:
content = file.read()
Dataclasses
Dataclasses are a new feature in Python 3.7 that provide a way to define classes with a minimal amount of code. For example, the following code defines a dataclass for a student:
from dataclasses import dataclass
@dataclass
class Student:
name: str
age: int
student1 = Student("John Doe", 20)
print(student1.name)
print(student1.age)
This code will print the following output:
John Doe 20
Dataclasses are a great way to write more concise and readable code.
These are just a few of the many easy Python features that you can start using today to write better code. I encourage you to experiment with these features and see how they can help you improve your code.
What are key features of Python?
Python is a general-purpose, high-level programming language. It is one of the most popular programming languages in the world, and it is used for a wide variety of tasks, including:
- Web development
- Data science
- Machine learning
- Artificial intelligence
- Scientific computing
- Software development
Python is known for its:
- Easy-to-learn syntax: Python has a very simple syntax that is easy to learn for beginners.
- Readability: Python code is very readable, which makes it easy to understand and maintain.
- Powerful: Python is a very powerful language that can be used to create complex applications.
- Open source: Python is an open source language, which means that it is free to use and modify.
- Large community: Python has a large and active community of developers who are constantly creating new libraries and tools.
These features, among others, contribute to Python’s popularity and versatility, making it an excellent choice for beginners and experienced developers alike.