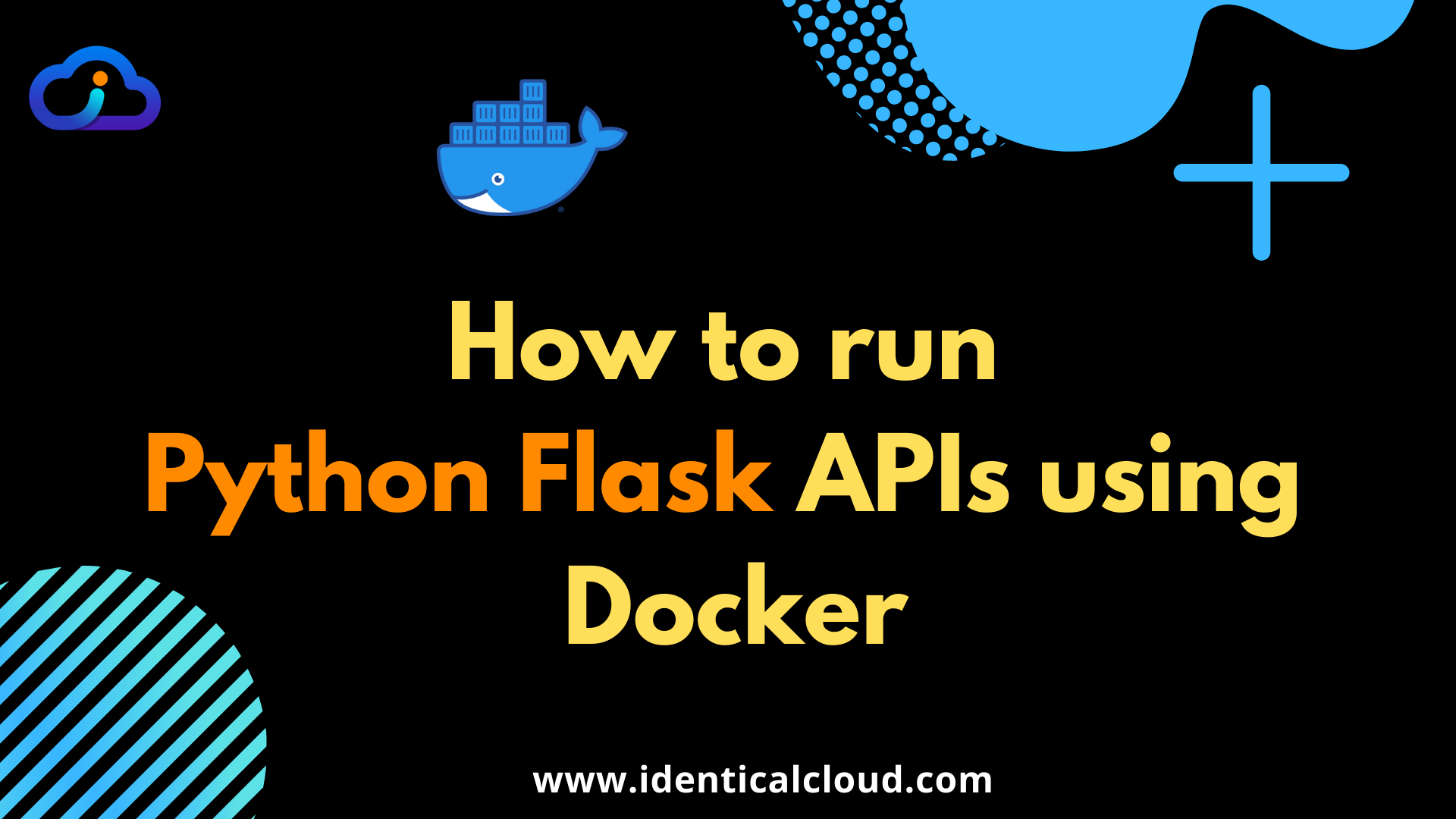
How to run Python Flask APIs using Docker
Python is a simple yet powerful coding language and it’s used widely in machine learning, artificial intelligence, and backend development. The reason why python is used widely is, its easy to learn syntax and debugging, since there are no compilation steps, it is easy to debug. Flask is a Python framework that makes it easier to develop backend or application code using python.
In this tutorial, we will see how to deploy Python Flask App using Docker, so you can easily transmit your working code in development, testing, staging, or production environment as well to a colleague’s or partner’s computer. Docker can save hours of time trying to deploy same code to another machine that is not compatible.
So Let’s get started
Prerequisite
- Make sure, Docker and Docker Compose are installed, if not click on the link to get steps
- Know Python version for your code
1. Go to your project
- Clone your project in the required directory inside the server or local system.
- Checkout required branch
2. Create Dockerfile
- Create new
Dockerfile
- Paste below-mentioned code
FROM python:3.6
WORKDIR /app
COPY requirement* requirement.txt
RUN pip install -r requirement.txt
COPY . .
EXPOSE 5000
CMD ["flask","run"]
Here,
- On line 1, Add Python version as per your requirement
- 2nd line defines working directory inside container, keep it as /app or define that custom
- 3rd line copies your requirements.txt file which has a list of packages that needs to be installed before running the code
- If you have a different file name for the packages file, change it accordingly
- 4th line will install all required packages as mentioned in the file copied in 3rd step
- 5th line will copy all your coding files from the current directory to /app working directory
- 6th line will expose your port number on which flask app is configured to run
- 7th line will tell your container at the time of startup to run
flask run
command
Here, you can define Environment variables in Dockerfile if required
3. Build Dockerfile
To run above Dockerfile
, run this command
docker build -t python-flask:1 .
where, python-flask
is your image name and 1
is image tag
You can change image tags when you create new revisions of your code
After successfully creating a Docker image out of your Dockerfile, Let’s run this Dockerfile and create a container
4. Deploy Docker image
docker run -d --name python-flask-app --restart always -p 5000:5000 python-flask:1
here -p contains 2 ports system port and container port,
If you are running some other code or service on 5000 port, you can change the system’s port and use any available port, that’s the flexibility Docker provides, where you don’t require to change the code to change the port number
For an e.g.
if 5000 port on the system is not available, you can change it via changing the first parameter -p 80:5000, it will start serving on the systemโs 80 port.
Now, this command will run your flask app.
You can check console logs of your application using below command
docker logs -f python-flask-app
It will stream all live logs from your python container
5. Automation
You can also automate this use case, using simple shell-script as below
python-deployment.sh
#!/bin/bash
docker build -t python-flask:latest .
docker rm -f python-flask-app || true
docker run -d --name python-flask-app --restart always -p 5000:5000 python-flask:latest
In above script,
- You can also create variable for the docker image tag, which can be defined run-time
- 1st command will build your Docker image with new code
- 2nd command will destroy the currently running container if exists and if the container doesn’t exist it will return true and move to the next step
- 3rd command will run your newly build docker image
6. Testing
To test it,
curl localhost:5000
curl $Elastic-IP:5000
You can use the curl command to fetch the response from your flask API, if it doesn’t respond you can check docker logs and troubleshoot the error.
7. Sharing Docker image
If you want to share this Docker image with your teammates or colleagues, simply upload it to Docker Images repositories from where it can be shared with others.
There are various Docker image repositories available
- Docker hub
- AWS ECR
- Google Container Registry
- Azure Container Registry
- Gitlab Container Registry
To know how to upload it to AWS ECR and Docker hub, follow provided links
And Done!!!
Your python Flask App is now Dockerized and ready with automation script.
If you like this article share your views in the comment section below.
Do share it if you find it useful
Drafted On,
May 22nd, 2021
DevOps @ identicalCloud.com
References
[1] Docker
[2] Install Docker and Docker Compose
[3] How to push and pull private docker images on Docker Hub
[4] How to push and pull private docker images on AWS ECR