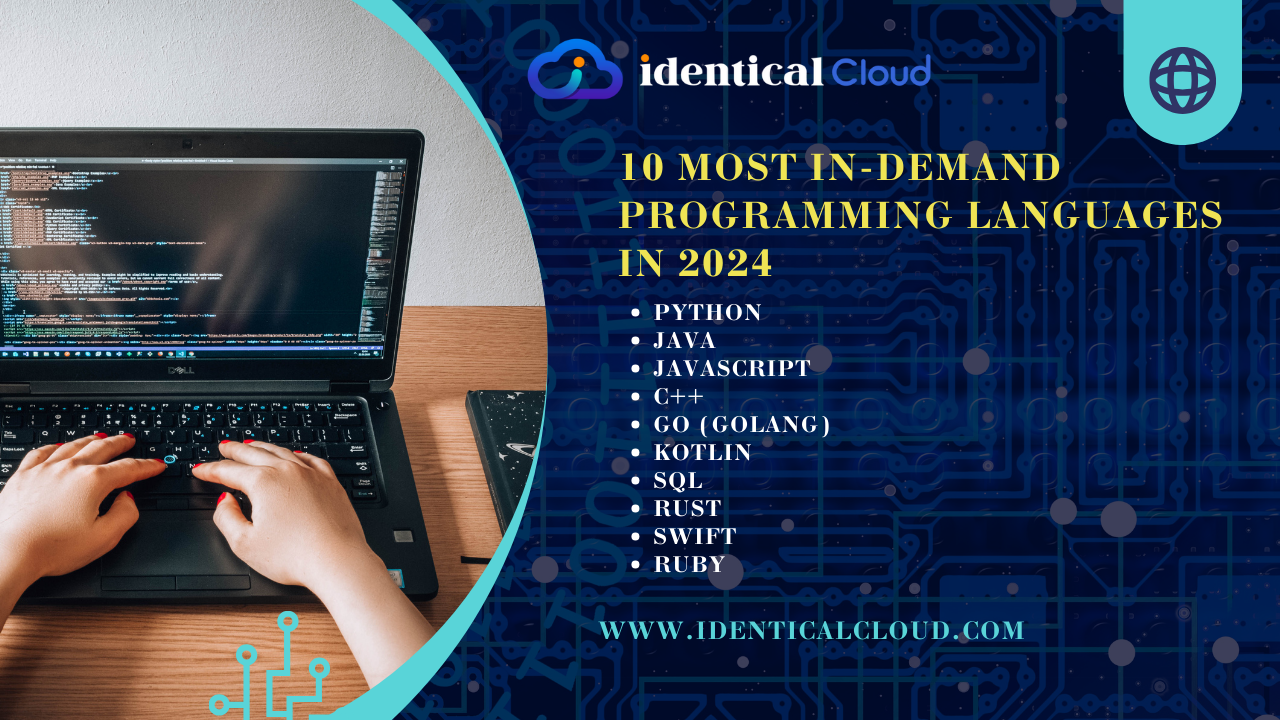
10 Most In-Demand Programming Languages in 2024
10 Most In-Demand Programming Languages in 2024
The world of programming is constantly evolving, with new languages and technologies emerging all the time. However, some languages remain consistently in demand, due to their versatility, performance, and popularity.
Here is a list of the 10 most in-demand programming languages in 2024:
Python
Python is a general-purpose programming language that is known for its simplicity and readability. It is widely used for web development, data science, and machine learning. Python is also a popular language for teaching programming, as it is relatively easy to learn.
Python has a number of features that make it a good choice for a variety of tasks. It is a dynamically typed language, which means that the type of a variable is not determined until the program is running. This makes Python code more flexible and easier to write. Python also has a large and active community, which means that there are many resources available to help users learn and use the language.
To write Python code, you can use any text editor, but there are also many IDEs (integrated development environments) available that are specifically designed for Python programming. Some popular IDEs for Python include PyCharm, Spyder, and Visual Studio Code.
Once you have chosen an editor or IDE, you can start writing your code. Python code is written in plain text files with a .py
extension.
To write a simple Python program, you can start by creating a new file and saving it with a .py
extension. Then, you can start writing your code in the file.
For example, the following Python code will print “Hello, world!” to the console:
print("Hello, world!")
To run this code, you can save the file and then run it in your editor or IDE.
Python code is made up of statements and expressions. Statements are instructions that tell Python what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the print()
statement in the example above, or they can be more complex, such as conditional statements (if
, elif
, and else
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (my_function(10, 5)
)
Python code is organized into blocks. Blocks are groups of statements that are indented together. Indentation tells Python which statements belong to the same block.
For example, the following Python code defines a function called my_function()
:
def my_function(a, b):
"""This function returns the sum of two numbers."""
return a + b
The body of the function is indented by four spaces. This tells Python that all of the statements inside the function block belong to the function.
To call the my_function()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the my_function()
function with the arguments 10
and 5
:
result = my_function(10, 5)
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
Python is a powerful and versatile programming language that can be used to create a wide variety of applications. If you are interested in learning more about Python, there are many resources available online and in libraries.
Java
Java is a general-purpose programming language that is known for its reliability and security. It is widely used for enterprise applications, mobile apps, and cloud computing.
To write Java code, you can use any text editor, but there are also many IDEs (integrated development environments) available that are specifically designed for Java programming. Some popular IDEs for Java include Eclipse, IntelliJ IDEA, and NetBeans.
Once you have chosen an editor or IDE, you can start writing your code. Java code is written in plain text files with a .java
extension.
To write a simple Java program, you can start by creating a new file and saving it with a .java
extension. Then, you can start writing your code in the file.
For example, the following Java code will print “Hello, world!” to the console:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, world!");
}
}
To run this code, you will need to compile it into a bytecode file. You can do this using the Java compiler, which is included in the Java SDK.
Once the code has been compiled, you can run it using the Java Virtual Machine (JVM). The JVM is a program that executes Java bytecode files.
To run the Java program above, you would open a terminal window and navigate to the directory where the compiled bytecode file is located. Then, you would type the following command:
java HelloWorld
This would start the JVM and execute the HelloWorld
program.
Java code is made up of classes and methods. Classes are blueprints for objects, and methods are functions that belong to classes.
The main method in the HelloWorld
program above is a special method that is called when the program is run. The main()
method usually contains the code that the program executes.
To write more complex Java programs, you will need to learn about classes, objects, and methods. There are many resources available online and in libraries that can teach you about these concepts.
Java is a powerful and versatile programming language that can be used to create a wide variety of applications. If you are interested in learning more about Java, there are many resources available online and in libraries.
JavaScript
JavaScript is a front-end programming language that is used to create interactive web pages. It is also increasingly being used for back-end development and serverless computing.
To write JavaScript, you will need to create a new file with a .js
extension. Then, you can start writing your code in the file.
Here is a simple JavaScript program that prints “Hello, world!” to the console:
console.log("Hello, world!");
To run this code, you can use a web browser or a JavaScript interpreter.
If you are using a web browser, you can create a new HTML file and add the following code to the <head>
section:
<script src="hello-world.js"></script>
Then, you can open the HTML file in your web browser and the “Hello, world!” message will be printed to the console.
If you are using a JavaScript interpreter, you can type the following command in the terminal:
node hello-world.js
This will run the JavaScript program and print the “Hello, world!” message to the console.
JavaScript code is made up of statements and expressions. Statements are instructions that tell JavaScript what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the console.log()
statement in the example above, or they can be more complex, such as conditional statements (if
, elif
, and else
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (myFunction(10, 5)
)
JavaScript code is organized into blocks. Blocks are groups of statements that are indented together. Indentation tells JavaScript which statements belong to the same block.
For example, the following JavaScript code defines a function called myFunction()
:
function myFunction(a, b) {
// This function returns the sum of two numbers.
return a + b;
}
The body of the function is indented by four spaces. This tells JavaScript that all of the statements inside the function block belong to the function.
To call the myFunction()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the myFunction()
function with the arguments 10
and 5
:
const result = myFunction(10, 5);
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
JavaScript is a powerful and versatile programming language that can be used to create a wide variety of applications, including web pages, web applications, and mobile applications.
C++
C++ is a high-performance programming language that is known for its speed and efficiency. It is widely used for system programming, game development, and scientific computing.
To write C++, you will need to create a new file with a .cpp
extension. Then, you can start writing your code in the file.
Here is a simple C++ program that prints “Hello, world!” to the console:
#include <iostream>
int main() {
std::cout << "Hello, world!" << std::endl;
return 0;
}
To compile and run this code, you will need a C++ compiler. There are many free and open source C++ compilers available, such as GCC and Clang.
Once you have installed a C++ compiler, you can compile the code above using the following command:
g++ hello_world.cpp -o hello_world
This will create an executable file called hello_world
. You can then run the program using the following command:
./hello_world
This will print the message “Hello, world!” to the console.
C++ code is made up of statements and expressions. Statements are instructions that tell the compiler what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the std::cout << "Hello, world!" << std::endl;
statement in the example above, or they can be more complex, such as conditional statements (if
, elif
, and else
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (myFunction(10, 5)
)
C++ code is organized into blocks. Blocks are groups of statements that are enclosed in curly braces ({}
). Blocks are used to group related statements together and to control the flow of execution of the program.
For example, the following C++ code defines a function called myFunction()
:
int myFunction(int a, int b) {
// This function returns the sum of two numbers.
return a + b;
}
The body of the function is enclosed in curly braces. This tells the compiler that all of the statements inside the function block belong to the function.
To call the myFunction()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the myFunction()
function with the arguments 10
and 5
:
int result = myFunction(10, 5);
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
C++ is a powerful and versatile programming language that can be used to create a wide variety of applications, including operating systems, games, and scientific software.
Go (Golang)
Go is a general-purpose programming language that is known for its simplicity, concurrency, and performance. It is widely used for web servers, cloud computing applications, and microservices.
To write Go code, you will need to create a new file with a .go
extension. Then, you can start writing your code in the file.
Here is a simple Go program that prints “Hello, world!” to the console:
package main
import "fmt"
func main() {
fmt.Println("Hello, world!")
}
To compile and run this code, you will need the Go compiler and the Go runtime installed on your system. You can download the Go compiler and runtime from the official Go website.
Once you have installed the Go compiler and runtime, you can compile the code above using the following command:
go build hello_world.go
This will create an executable file called hello_world
. You can then run the program using the following command:
./hello_world
This will print the message “Hello, world!” to the console.
Go code is made up of packages, packages are groups of related Go files. The package
statement at the top of the file tells the Go compiler which package the file belongs to.
Go code is also made up of functions. A function is a block of code that performs a specific task. The func
statement tells the Go compiler that the following block of code is a function.
The main()
function is a special function that is called when the program is run.
Go code is also made up of statements and expressions. Statements are instructions that tell the Go compiler what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the fmt.Println("Hello, world!")
statement in the example above, or they can be more complex, such as conditional statements (if
, else
, and switch
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (myFunction(10, 5)
)
Go code is organized into blocks. Blocks are groups of statements that are enclosed in curly braces ({}
). Blocks are used to group related statements together and to control the flow of execution of the program.
For example, the following Go code defines a function called myFunction()
:
func myFunction(a, b int) int {
// This function returns the sum of two numbers.
return a + b
}
The body of the function is enclosed in curly braces. This tells the Go compiler that all of the statements inside the function block belong to the function.
To call the myFunction()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the myFunction()
function with the arguments 10
and 5
:
result := myFunction(10, 5)
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
Go is a powerful and versatile programming language that can be used to create a wide variety of applications, including web servers, command-line tools, and distributed systems.
Kotlin
Kotlin is a modern programming language that is fully interoperable with Java. It is known for its concise syntax and expressive features. Kotlin is becoming increasingly popular at Google, especially for Android development.
To write Kotlin code, you will need to create a new file with a .kt
extension. Then, you can start writing your code in the file.
Here is a simple Kotlin program that prints “Hello, world!” to the console:
fun main(args: Array<String>) {
println("Hello, world!")
}
To compile and run this code, you will need the Kotlin compiler installed on your system. You can download the Kotlin compiler from the official Kotlin website.
Once you have installed the Kotlin compiler, you can compile the code above using the following command:
kotlinc hello_world.kt
This will create an executable file called hello_world.jar
. You can then run the program using the following command:
java -jar hello_world.jar
This will print the message “Hello, world!” to the console.
Kotlin code is made up of functions, classes, and objects. A function is a block of code that performs a specific task. A class is a blueprint for creating objects. An object is an instance of a class.
The main()
function is a special function that is called when the program is run.
Kotlin code is also made up of statements and expressions. Statements are instructions that tell the Kotlin compiler what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the println("Hello, world!")
statement in the example above, or they can be more complex, such as conditional statements (if
, else
, and when
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (myFunction(10, 5)
)
Kotlin code is organized into blocks. Blocks are groups of statements that are indented together. Indentation tells the Kotlin compiler which statements belong to the same block.
For example, the following Kotlin code defines a function called myFunction()
:
fun myFunction(a: Int, b: Int): Int {
// This function returns the sum of two numbers.
return a + b
}
The body of the function is indented by four spaces. This tells the Kotlin compiler that all of the statements inside the function block belong to the function.
To call the myFunction()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the myFunction()
function with the arguments 10
and 5
:
val result = myFunction(10, 5)
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
Kotlin is a powerful and versatile programming language that can be used to create a wide variety of applications, including Android apps, web apps, and server-side applications.
SQL
SQL is a programming language that is used to communicate with databases. It is used to create, read, update, and delete data in databases. SQL is a declarative language, which means that you tell the database what you want to do, but not how to do it. The database optimizer is responsible for figuring out the most efficient way to execute your query.
To write SQL code, you will need to use a SQL client. A SQL client is a tool that allows you to interact with a SQL database. There are many different SQL clients available, both free and commercial.
Once you have installed a SQL client, you can connect to your database and start writing SQL code.
Here is a simple SQL query that selects all of the data from the employees
table:
SELECT * FROM employees;
This query will return all of the rows in the employees
table, along with all of the columns in each row.
You can also use SQL to filter the data that is returned by a query. For example, the following query will select all of the employees in the employees
table who have a salary greater than $100,000:
SELECT * FROM employees WHERE salary > 100000;
You can also use SQL to group and aggregate data. For example, the following query will group the employees in the employees
table by department and calculate the average salary for each department:
SELECT department, AVG(salary) AS avg_salary FROM employees GROUP BY department;
This query will return a table with two columns: department
and avg_salary
. The department
column will contain the name of each department, and the avg_salary
column will contain the average salary for each department.
SQL is a powerful language that can be used to perform a wide variety of tasks on a SQL database. If you are interested in learning more about SQL, there are many resources available online and in libraries.
Here is an example of a more complex SQL query:
SELECT name, department, salary
FROM employees
JOIN departments ON employees.department_id = departments.department_id
WHERE departments.name = 'Engineering' AND salary > 100000;
This query will join the employees
and departments
tables on the department_id
column. This will allow us to filter the results to only include employees who work in the Engineering department and have a salary greater than $100,000.
SQL is a powerful tool for data analysis and manipulation. It is used by database administrators, data analysts, and developers to manage and interact with data in relational databases.
Rust
Rust is a multi-paradigm, general-purpose programming language designed for performance and safety, especially safe concurrency. Rust is syntactically similar to C, but can guarantee memory safety by values being checked at compile time. It has a rich type system with ownership and borrowing, which allows developers to write safe code without having to rely on garbage collection.
To write Rust code, you will need to create a new file with a .rs
extension. Then, you can start writing your code in the file.
Here is a simple Rust program that prints “Hello, world!” to the console:
fn main() {
println!("Hello, world!");
}
To compile and run this code, you will need the Rust compiler installed on your system. You can download the Rust compiler from the official Rust website.
Once you have installed the Rust compiler, you can compile the code above using the following command:
rustc hello_world.rs
This will create an executable file called hello_world
. You can then run the program using the following command:
./hello_world
This will print the message “Hello, world!” to the console.
Rust code is made up of functions, modules, and structs. A function is a block of code that performs a specific task. A module is a way to organize Rust code into logical groups. A struct is a data structure that can be used to store and manipulate data.
The main()
function is a special function that is called when the program is run.
Rust code is also made up of statements and expressions. Statements are instructions that tell the Rust compiler what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the println!("Hello, world!");
statement in the example above, or they can be more complex, such as conditional statements (if
, else
, and match
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (my_function(10, 5)
)
Rust code is organized into blocks. Blocks are groups of statements that are enclosed in curly braces ({}
). Blocks are used to group related statements together and to control the flow of execution of the program.
For example, the following Rust code defines a function called my_function()
:
fn my_function(a: i32, b: i32) -> i32 {
// This function returns the sum of two numbers.
return a + b;
}
The body of the function is enclosed in curly braces. This tells the Rust compiler that all of the statements inside the function block belong to the function.
To call the my_function()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the my_function()
function with the arguments 10
and 5
:
let result = my_function(10, 5);
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
Rust is a powerful and versatile programming language that can be used to create a wide variety of applications, including web servers, command-line tools, and embedded systems.
Swift
Swift is a general-purpose, multi-paradigm, compiled programming language developed by Apple Inc. and the open-source community. First released in 2014 as a successor to the Objective-C language, it is used to develop iOS, iPadOS, macOS, watchOS, tvOS, and Linux applications.
To write Swift code, you will need to create a new file with a .swift
extension. Then, you can start writing your code in the file.
Here is a simple Swift program that prints “Hello, world!” to the console:
print("Hello, world!")
To compile and run this code, you will need the Swift compiler installed on your system. You can download the Swift compiler from the official Apple website.
Once you have installed the Swift compiler, you can compile the code above using the following command:
swiftc hello_world.swift
This will create an executable file called hello_world
. You can then run the program using the following command:
./hello_world
This will print the message “Hello, world!” to the console.
Swift code is made up of functions, classes, and structs. A function is a block of code that performs a specific task. A class is a blueprint for creating objects. A struct is a data structure that can be used to store and manipulate data.
The print()
function is a special function that prints the specified text to the console.
Swift code is also made up of statements and expressions. Statements are instructions that tell the Swift compiler what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the print("Hello, world!")
statement in the example above, or they can be more complex, such as conditional statements (if
, else
, and switch
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (myFunction(10, 5)
)
Swift code is organized into blocks. Blocks are groups of statements that are indented together. Indentation tells the Swift compiler which statements belong to the same block.
For example, the following Swift code defines a function called myFunction()
:
func myFunction(a: Int, b: Int) -> Int {
// This function returns the sum of two numbers.
return a + b
}
The body of the function is indented by four spaces. This tells the Swift compiler that all of the statements inside the function block belong to the function.
To call the myFunction()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the myFunction()
function with the arguments 10
and 5
:
let result = myFunction(a: 10, b: 5)
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
Ruby
Ruby is a general-purpose, dynamic, reflective, object-oriented programming language designed and developed in the mid-1990s by Yukihiro “Matz” Matsumoto in Japan. It was first distributed outside of Japan in 1995.
To write Ruby code, you will need to create a new file with a .rb
extension. Then, you can start writing your code in the file.
Here is a simple Ruby program that prints “Hello, world!” to the console:
puts "Hello, world!"
To run this code, you can use a Ruby interpreter. There are many different Ruby interpreters available, both free and commercial.
Once you have installed a Ruby interpreter, you can run the code above using the following command:
ruby hello_world.rb
This will print the message “Hello, world!” to the console.
Ruby code is made up of statements and expressions. Statements are instructions that tell the Ruby interpreter what to do, and expressions are pieces of code that evaluate to a value.
Statements can be simple, such as the puts "Hello, world!"
statement in the example above, or they can be more complex, such as conditional statements (if
, else
, and unless
) and loops (for
and while
).
Expressions can be simple, such as the number 10
, or they can be more complex, such as mathematical expressions (10 + 5 * 2
) and function calls (my_function(10, 5)
)
Ruby code is organized into blocks. Blocks are groups of statements that are indented together. Indentation tells the Ruby interpreter which statements belong to the same block.
For example, the following Ruby code defines a function called my_function()
:
def my_function(a, b)
# This function returns the sum of two numbers.
return a + b
end
The body of the function is indented by four spaces. This tells the Ruby interpreter that all of the statements inside the function block belong to the function.
To call the my_function()
function, you would simply write the function name followed by parentheses, and then pass in the arguments that the function requires. For example, the following code would call the my_function()
function with the arguments 10
and 5
:
result = my_function(10, 5)
The variable result
would then contain the value 15
, which is the sum of the two arguments passed to the function.
Ruby is a powerful and versatile programming language that can be used to create a wide variety of applications, including web applications, command-line tools, and desktop applications.
Here is an example of a more complex Ruby program:
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
def greet
"Hello, my name is #{@name} and I am #{@age} years old."
end
end
person = Person.new("Alice", 25)
puts person.greet
This program defines a class called Person
with two attributes: name
and age
. The class also has a method called greet()
, which returns a string that greets the user.
On the last line of the program, we create a new instance of the Person
class and assign it to the variable person
. We then call the greet()
method on the person
object and print the result to the console.
This example shows how Ruby can be used to create classes and objects to model real-world entities.
These languages are all in high demand due to their versatility, performance, and popularity. If you are interested in learning a new programming language, or if you are looking for a language that will give you a competitive edge in the job market, any of these 10 languages would be a great choice.
Why is it important to learn a programming language?
There are many reasons why it is important to learn a programming language. Programming languages allow you to create software applications, websites, and other digital products. They also allow you to automate tasks and create scripts to improve your productivity.
In addition, programming languages are in high demand in the job market. Many employers are looking for employees with programming skills, and salaries for programmers are typically higher than average.
How to choose the right programming language for you
When choosing a programming language to learn, there are a few factors you should consider:
- Your interests:ย What kind of software or applications do you want to create? Choose a language that is well-suited for your interests.
- Your career goals:ย If you are interested in a career in software development, choose a language that is in high demand in the job market.
- Your learning style: Some programming languages are easier to learn than others. Choose a language that is appropriate for your learning style.
Remember that the programming languages in demand can vary depending on your location, industry, and specific job market conditions. To ensure you stay competitive in 2024, consider staying up-to-date with emerging technologies and trends in software development while mastering the fundamentals of these languages.